Purpose of the Article: The purpose of this article is to help Angular Developers to Manage State in Angular Applications.
Intended Audience: Angular Developers.
Tools and Technology: Angular, NgRx, RxJS.
Keywords: NgRx, State-Management.
Table of Contents
- State Management
- NgRx
- NgRx Lifecycle
- NgRx Implementation
Prerequisites
- Angular 12
- RxJS
- Typescript
State Management
- What is the State? The State is part of application data, such as the list of employees, etc
- What is Application State? It is the whole data of the application, i.e., data received via API calls from the database. We maintain the entire application state in-store using NgRx
- What is State Management? It is the synchronization of application’s data throughout all components and services of the application. It provides a clear picture of the pplication state at any given moment
Advantages of State Management
- The whole application state is available in one place
- Using NgRx in angular reduces the API calls for retrieval of the data. If any modification is done in a state or new data is added, send an HTTP request to the server
- State management helps to maintain the application code very quickly
- State management improves the code quality by reducing the code size and making it more readable
- Local storage sync with the application helps retain the data whenever you refresh the page
NgRx
- NgRx stands for Angular Reactive Extensions. It is a framework for developing reactive applications and is an open-source library that provides state management for your angular applications
- NgRx/store implements the redux pattern using RxJS observables
- NgRx maintains the whole application state in a single place called a store and uses streams to interact with the store
- No need to inject multiple services into components to manage communication between them. NgRx contains the whole application state from a single source
- NgRx simplified the process of data management in the angular application. Components and services connect to NgRx/store to get the active state of the application state and state manipulation
Flow of application state in NgRx
NgRx Lifecycle:/strong>
- NgRx comprises five main concepts: store, actions, reducers, selectors, and effects
Store
- You can think it is a client-side database
- It holds up the application’s current state
Actions
- Actions describe how the state should be changed
- Actions are one of the main building blocks in NgRx
- For example, adding an employee can be an action that will change the state (i.e., add a new employee to the list)
- Actions describe how the state should be changed
Reducer
- Reducers are pure functions responsible for handling transitions from one state to the next in your application.
- Reducers receive the action and state and respond based on the activity dispatched. It implements the logic and updates the store with a new state
- Reducers take the latest action dispatched to the current state and determine whether to return a newly modified state or the original state
Selector
- What is Selector? Selectors are pure functions
- Selectors are used to retrieving a part of the application state from the store
Effects
- Effects deal with side-effects caused by dispatching an action outside the NgRx store
- Effects used to get or send data to an API
- Effects also trigger actions based on the API responses (success, error, etc.) to update the part of the State.
Data flow in NgRx
NgRx Implementation
I have created a demo application with the basic structure and components to help you implement the NgRx-related part in angular.
Required Npm packages to work with NgRx
How to configure reducer and effects in App.Module.ts
Create one employee model
- ng g class Employee –type =model
employee. model.ts file
Create One Service
command: ng g s employee
employee. service.ts file
Create a folder named store and add all the store-related files.
- Let’s start NgRx implementation by adding a file name employee.action.ts
- Create employee. reducers.ts file
- Create employee. effects.ts file
- Create employee. selector.ts file
Create component employee
- command: ng g c employee
employee.component.html file
employee. component.ts file
Create one component to deal with the editing and insertion of the employee
- Command: ng g c add-employee
Add-employee.html file
Add-employee.ts file
Output
Employees list
Employee insertion
Employee update
Thanks & Regards,
Shireesha BHUMIREDDY
Software Engineer
Enterprise application Development- Digital Transformation
- shireeshab.in@mouritech.com
Author Bio:
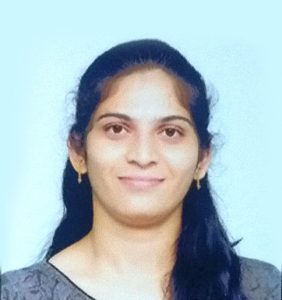
Shireesha BHUMIREDDY
Enterprise Application Development- Digital Transformation
Angular developer with over 2 years of experience in software development. Passionate about developing user-friendly web applications. Proven ability to work independently and as part of a team.