Purpose of the article: This article provides a comprehensive guide to implementing coding standards and best practices for writing maintainable React code. It covers essential topics such as folder structure, naming conventions, component structure, state management, styling, and code quality. By following these coding standards, development teams can build React applications that are scalable, readable, and much easier to maintain over the long term. The presentation will provide developers with both high-level concepts and concrete examples that can be applied to projects today.
Intended Audience: Frontend developers (ReactJS)
Tools and Technology: ReactJS
Please go through the first part of the article – https://www.mouritech.com/it-technical-articles/crafting-maintainable-react-code-a-guide-to-react-coding-standards/
State Management
For managing state in larger apps, React projects typically use a third-party state management library like Redux or MobX.
Here are some tips for keeping state management code clean:
Structure reducers by domain: Split reducer logic based on features/domains vs actions.
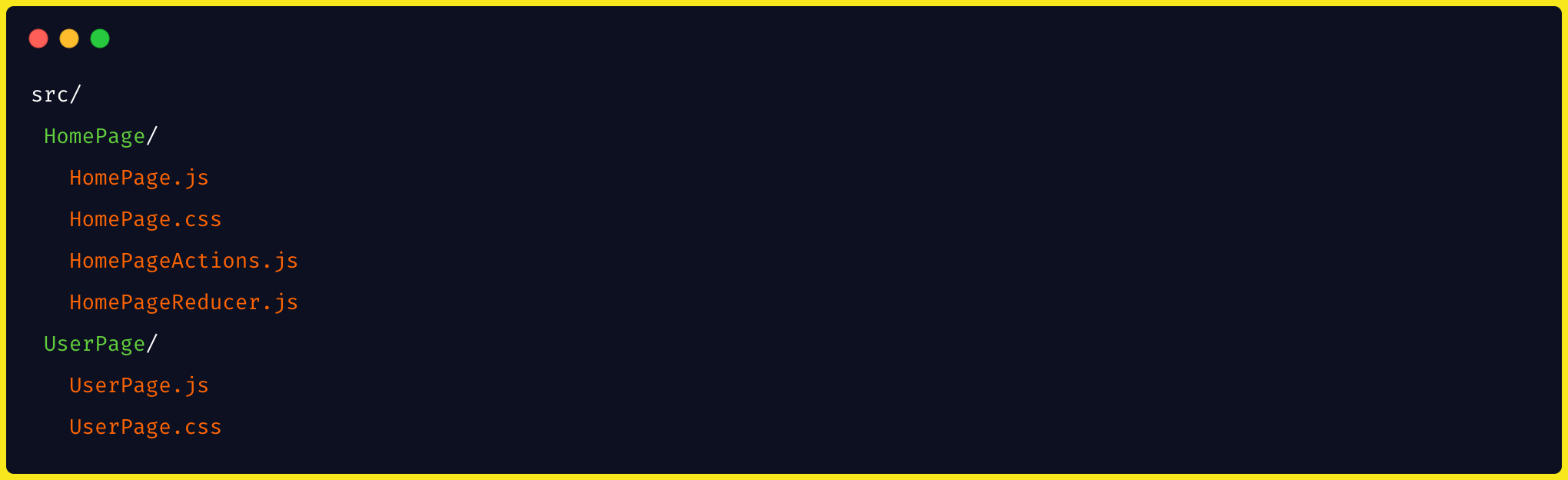
Create Slice Reducers: createSlice() generates action creators and reducers

Use selector functions: Selectors extract/transform data from state.

CreateAsyncThunk: abstracts async logic into easy to use actions

Manage Async State: The extraReducers builder handles async status automatically

Keeping state logic organized makes it much easier to trace and debug.
Styling
There are several ways to handle styling in React apps – Here’s more on React styling best practices:
– CSS Modules: CSS Modules scope styles locally and prevent clashes.
– Avoid global styles: Use component-specific CSS Modules instead of global CSS files.
– Use utility classes: Utilities like Tailwind provide low-level reusable styles.
– Style components directly: For one-off styles, use the style prop.
– Extract reusable styles: Move repeating styles into shared CSS Modules.
– Name classes by component: Prefix CSS classes with the component name.
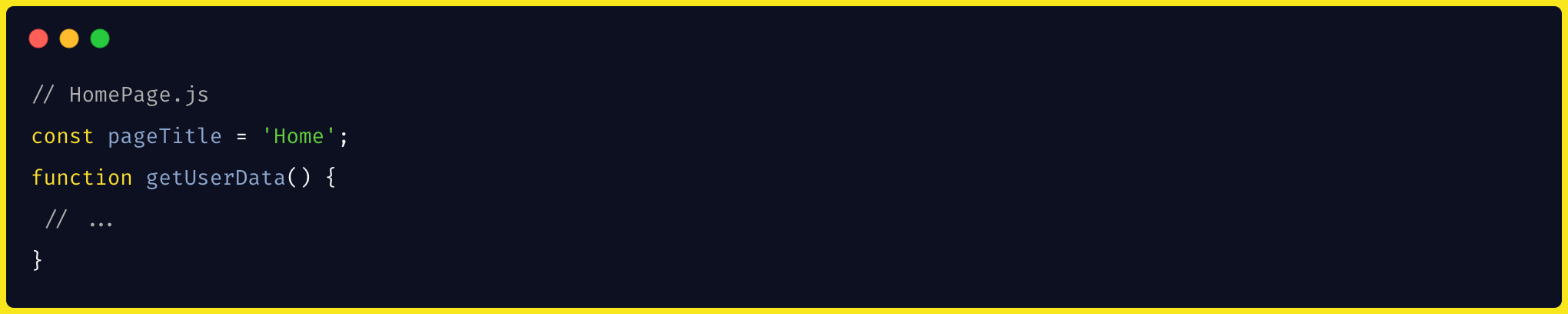
– Use variables for colors, etc: Store common values like colors and fonts in variables.
– Avoid nested selectors: Nesting CSS selectors tightly couples style to structure.
– Use BEM for block/element modifiers: BEM provides a perfect way to modify styles.

Keeping styles modular, reusable, and loosely coupled helps prevent a tangled mess of CSS.
Code Quality
Here are some other tips for writing clean React code:
- Lint and format code: Use ESLint and Prettier to enforce consistent conventions.
- Write small functions: Break code into smaller single-purpose functions.
- Avoid duplication: Factor out any duplicate code into reusable functions/components.
- Use immutable data: Immutable data makes logic easier to reason about, so put it to use.
- Validate props: Use PropTypes to validate components are passed to correct props.
- Add comments for complex code: Comments explain the why – when code isn’t obvious.
- Delete unused code: Remove any unused components, utilities, etc.
- Use TypeScript: TypeScript can help catch bugs and thus improve readability.
Testing
Testing is critical for preventing regressions and building maintainable React components:
- Unit test components: Jest, React Testing Library and Enzyme allow unit testing components.
- Mock dependencies: Mock any APIs, global state etc.
- Test edge cases: Validate error states and edge cases.
- Use snapshot tests: Snapshot tests help prevent unintended changes.
- Test on real browsers: Cypress and Selenium provide browser-based testing.
- Test accessibility: Validate screen reader accessibility with tools like React-axe.
- Set up CI: Run tests on every commit with CI like GitHub Actions.
Conclusion
Crafting maintainable React code requires forethought and discipline. By leveraging conventions like consistent structure, modular components, and clear naming, you can create React apps that are a joy to work. Automated tools like linters, tests, and TypeScript also complement these best practices.
With these standards in place, developers can focus their energy on shipping features rather than struggling with a tangled mess of code. The end result is happy developers and performant applications.
References:
- https://legacy.reactjs.org/docs/design-principles.html
- https://redux-toolkit.js.org/usage/usage-guide
- https://engineering.hmn.md/standards/style/react
- https://www.dhiwise.com/post/unleashing-the-potential-of-react-eslint-an-essential-guide
- https://blog.bitsrc.io/react-philosophy-for-beginners-d926a7672e66
Author Bio:
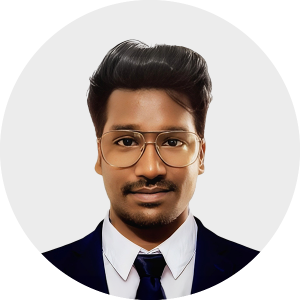
Yagnya Mohan Kumar BONTHU
Senior Software Engineer - Enterprise Architecture-Digital Transformation
A passionate software engineer with over 4+ years of experience developing web and mobile applications. Skilled in front-end and back-end technologies and loves to create intuitive, pixel-perfect user interfaces that provide great experience for users.