Purpose of the article: “Enhance User Experience with Particle Effects in Angular” is a comprehensive guide that explores the integration of particle effects into your Angular applications using the @tsparticles/angular library. This article provides readers with a step-by-step tutorial on how to add visually appealing and engaging particle effects to their applications. It covers everything from setting up the Angular project and installing the necessary libraries to configuring particles directly in the component or via a JSON file. Whether you’re a web developer or an Angular enthusiast, this article equips you with the knowledge to effortlessly incorporate dynamic particle effects into your digital projects, enhancing user engagement and visual appeal.
Intended Audience: This guide is designed for web and Angular developers, UI/UX designers, and front-end developers looking to enhance their Angular applications with engaging visual effects. It provides step-by-step instructions, practical examples, and valuable insights for incorporating particle effects to elevate the user experience and interactivity of web applications.
Tools and Technology: HTML, Type script, Angular, `@tsparticles/angular `.
Keywords: Angular, Particles.js, Visual Effects, Front-End Development, UI/UX Design, Web Development.
Enhance User Experience with Particle Effects in Angular
Enhancing your Angular application with particle effects can greatly boost its visual appeal and create a more immersive user experience. This guide will lead you through the process of integrating @tsparticles/angular into your Angular application, presenting two approaches: direct configuration and configuration via a JSON file.
Why Use Particles in Angular?
Visual Appeal: Particle effects have the power to enhance the visual appeal of your application, giving it a more modern and captivating look.
Engagement: Dynamic backgrounds can keep users engaged and improve the overall user experience.
Customization: Extensive configuration options empower you to customize the particle effects to suit your preferences precisely.
- Need to install Particles library using this command:
npm install @tsparticles/angular @tsparticles/engine
Reference Link: https://www.npmjs.com/package/@tsparticles/angular?activeTab=readme
- Using loadSlim
To install the `loadSlim` package, execute the following command:
npm i @tsparticles/slim
If you need a specific version, use:
npm i @tsparticles/slim@version_number
Reference Link: https://www.npmjs.com/package/@tsparticles/slim?activeTab=versions
Implementation Methods:
Method 1: Direct Configuration
In the HTML file:
In the TypeScript file:
Import the required packages :
import { Component, OnInit } from '@angular/core';
import { RouterLink } from '@angular/router';
import { NgxParticles Module } from '@tsparticles/angular';
import { Container, tsParticles } from '@tsparticles/engine';
import { loadSlim} from '@tsparticles/slim';
@Component(
selector: app-home ',
standalone: true,
imports: [RouterLink, NgxParticlesModule],
templateUrl: './home.component.html',
styleUrls: ['/home.component.css'],
})
export class HomeComponent implements OnInit {
id= 'tsparticles';
particlesOptions: any;
constructor(private readonly ngParticlesService: NgParticlesService) {}
async ngOnInit(): Promise {
await loadSlim(tsParticles);
const configs = {
fpsLimit: 60,
particles: {
color: {
value: ['#E3F8FF', '#28CC9E', '#A6ED8E'],
},
move: {...
},
number: {...
},
opacity: {...
},
shape: {...
},
size: {...
},
},
background: {"
},
poisson: { "..
},
};
this.particlesOptions = {
background: {
color: {
value: configs.background.color,
},
},
particles: {
number: {
value: configs.particles.number.value,
},
color: {...
},
shape: {...
},
opacity: {"
},
size: {"
},
move: {***
},
},
interactivity: {"
},
retina_detect: true,
},
await tsParticles.load({
id: this.id,
options: this.particlesOptions,
});
}
particlesLoaded (container: Container): void {
console.log(container);
}
}
Through this link we need to change particles. In this link some examples are provided:
Reference Link: https://codepen.io/collection/DPOage
Method 2: Configuration via JSON File
In the HTML file:
In .ts file:
If we can achieve by a second way of implementation, we need to create a file in .json format. Provide file path in .ts file.
The ‘particles’ object needs to be included in the .json file.
export class HomeComponent {
id = 'tsparticles';
particlesUrl = 'assets/particles.json';
}
constructor(private readonly ngParticlesService: NgParticles Service) {}
ngOnInit(): void {
this.ngParticlesService.init(async () => {
await loadslim(tsParticles);
await tsParticles.load({
id: 'tsparticles',
options: {
preset: 'bubbles',
},
});
});
}
particlesLoaded (container: Container): void {
console.log(container);
}
How loadSlim Works:
The loadSlim function loads a pre-configured set of particle options that include the most commonly used features. It is intended for use cases where you do not need the full range of particle effects offered by the tsparticles library, thus providing a more optimized and efficient setup.
Use Cases for loadSlim:
1) Performance Optimization:
- Smaller Bundle Size: By loading only the essential particle features, loadSlim reduces the bundle size of your Angular application.
- Faster Load Times: A smaller bundle size results in faster load times, which is crucial for improving user experience, especially in web applications where performance is a priority.
2) Resource-Constrained Environments:
- Low-End Devices: If your application is expected to run on low-end devices with limited resources, using the slim version ensures better performance and smoother animations.
- Mobile Applications: For mobile web applications, where resources are limited and performance is critical, loadSlim helps maintain a balance between visual appeal and application responsiveness.
3) Minimalistic Particle Effects:
Basic Animations: If your application only requires basic particle effects and does not need the full range of features offered by tsparticles, using loadSlim is an optimal choice.
Detailed Breakdown of particlesOptions Object:
The particlesOptions object is the main configuration object for setting up particle effects. Here are some key properties and their purposes:
particles: This property defines the behavior and appearance of the particles.
- number: Specifies the number of particles.
- value: The actual number of particles.
- density: Controls the density of particles within a certain area.
- enable: A Boolean indicating whether movement is enabled or disabled.
- value_area: The area in which the particles are distributed is referred to as the value area.
- color: Specifies the color of the particles.
- value: Specify the color value(s) for the particles. This can be a single color or an array of colors.
- shape: This parameter specifies the shape of the particles.
- type: Shape type (e.g., “circle”, “square”, “triangle”).
- opacity: Controls the transparency of the particles.
- value: Opacity level (0 to 1).
- random: Boolean to enable random opacity levels.
- size: Indicates the size of the particles.
- value: Size of the particles.
- random: Boolean to enable random sizes.
- line_linked: Manages the linking lines between particles.
- enable: Boolean to enable or disable linking lines.
- distance: Maximum distance between particles for a line to be drawn.
- color: Color of the linking lines.
- opacity: Opacity of the linking lines.
- width: Width of the linking lines.
- move: Controls the movement of particles.
- enable: Boolean indicating whether movement is enabled or disabled.
- speed: Speed of the particle movement.
- direction: Movement direction (e.g., “none,” “top,” “top-right”).
- random: A Boolean that, when enabled, allows for random movement directions.
- straight: Boolean to enable straight-line movement.
- out_mode: How particles behave when they reach the edge (e.g., “out”, “bounce”).
- bounce: Boolean to enable bouncing off edges.
- interactivity: This property controls how users can interact with the particles.
- detect_on: Determines where to detect interactivity (e.g., “canvas”).
- events: Defines the interactive events.
- onhover: Actions when the user hovers over the particles.
- enable: Boolean to enable or disable hover effects.
- mode: Mode of interaction (e.g., “repulse”, “grab”).
- onclick: When clicked: Actions triggered by user interaction with the particles.
- enable: Boolean to enable or disable click effects.
- mode: Mode of interaction (e.g., “push”, “remove”).
- resize: Boolean to enable or disable particle resizing on window resize.
- onhover: Actions when the user hovers over the particles.
- modes: Configurations for different interaction modes.
- repulse: Settings for the repulse mode.
- distance: Distance for repulse effect.
- duration: Duration of the repulse effect.
- push: Settings for the push mode.
- particles_nb: Number of particles to push.
- repulse: Settings for the repulse mode.
- retina_detect: Boolean to enable or disable retina display support, improving visual quality on high DPI screens.
Conclusion:
By following these steps, you can successfully integrate particle effects into your Angular application using @tsparticles/angular, creating a more dynamic and engaging user experience. Experiment with different configurations to find the perfect particle effect for your project.
Author Bio:
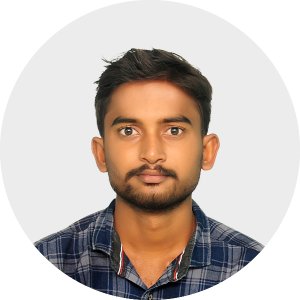
Matcha Viswanatha Kumar
Digital Transformation - Associate Software Engineer
I'm M. Viswanatha Kumar, a passionate software engineer specializing in UI development. With a Good knowledge of Java and extensive experience in building dynamic Angular applications, I focus on creating visually stunning and user-friendly interfaces. My expertise in front-end technologies allows me to craft seamless user experiences, blending functionality with aesthetic appeal. Constantly exploring the latest in web development, I bring innovation and precision to every project.