Purpose of the article: How we need to improve react native application performance in devices.
Intended Audience: React native developers
Tools and Technology: React native, Java script, and Typescript.
Keywords: React native application Performance improvement.
The Beginning
In the fast-paced world of software development today, making sure that applications are of good quality and reliable is the most important thing. Google’s open-source UI toolkit, Flutter, has gotten a lot of attention for making it easy to make apps that look great and work well on multiple devices. As a Flutter writer, it’s important to make sure that your apps are reliable, and writing test cases is a key part of that. The goal of this blog post is to give you a complete guide on how to write useful test cases in Flutter. This will allow you to make apps that are not only visually appealing but also well-tested and reliable.
Understanding Testing in Flutter
In Flutter, testing means making sure that your app works and behaves as expected so that you can find and fix any problems before releasing it. Writing well-organized test cases can help you in a number of important ways:
Early Bug Detection: Test cases help find bugs early in the development process, which saves time and resources compared to solving them after the fact.
This is because writing tests forces you to think about all the possible outcomes, which often results in code that is cleaner and easier to manage.
Higher Confidence: A strong test suite gives you peace of mind that your app will work as planned in a wide range of scenarios.
Unit Tests, Widget Tests, and Integration Tests are the three main types of test cases that are used in Flutter programming. Let’s look at each type in more depth and use real-life cases to help you understand better.
Widget Test
The purpose of widget tests is to check how different widgets or groups of widgets in your Flutter app look and behave. These tests act like a user interacting with the tools to make sure they look right and work as expected when different events happen or the state changes.
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build the widget tree
await tester.pumpWidget(const MyApp());
// Verify the initial state
expect(find.text('0'), findsOneWidget);
// Tap the '+' icon and trigger a frame
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that the counter incremented
expect(find.text('1'), findsOneWidget);
});
testWidgets('Counter decrements smoke test', (WidgetTester tester) async {
// Build the widget tree
await tester.pumpWidget(const MyApp());
// Set the initial counter value to 5
await tester.tap(find.byIcon(Icons.add)); // Tap 5 times
await tester.pump();
expect(find.text('5'), findsOneWidget);
// Tap the '-' icon and trigger a frame
await tester.tap(find.byIcon(Icons.remove));
await tester.pump();
// Verify that the counter decremented
expect(find.text('4'), findsOneWidget);
});
}
In this example, we test how a counter widget works by pretending to tap the “+” and “-” icons and making sure that the counter value goes up and down properly. We can set up and run widget tests with the help of the flutter_test package’s testWidgets function.
Unit Test
In Flutter, unit tests are a great way to look closely at separate pieces of code, like functions, methods, or classes. By focusing on single parts, developers can precisely test how they behave. Let’s look at a few examples of unit tests:
void main() {
test('String.trim() removes surrounding whitespace', () {
expect(' hello '.trim(), equals('hello'));
});
test('String.toUpperCase() converts a string to uppercase', () {
expect('hello'.toUpperCase(), equals('HELLO'));
});
}
Each of these examples checks the trim() and toUpperCase() methods of the String class on its own to make sure they work as intended.
Integration Test:
With integration tests, you can see how the different parts or sections of your Flutter app work together. These tests make sure that all of your app’s parts work together properly and can handle situations that happen in the real world.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
group('End-to-end test', () {
testWidgets('Login flow', (WidgetTester tester) async {
// Build the app
await tester.pumpWidget(const MyApp());
// Enter login credentials
await tester.enterText(find.byKey(const Key('emailField')), 'test@example.com');
await tester.enterText(find.byKey(const Key('passwordField')), 'password');
// Tap the login button
await tester.tap(find.byKey(const Key('loginButton')));
await tester.pumpAndSettle();
// Verify successful login
expect(find.byKey(const Key('homeScreen')), findsOneWidget);
// Simulate logout
await tester.tap(find.byKey(const Key('logoutButton')));
await tester.pumpAndSettle();
// Verify user is navigated back to login screen
expect(find.byKey(const Key('loginScreen')), findsOneWidget);
});
});
}
In this example, we test an app’s login flow by pretending to be a user, entering login information, and making sure that the user is taken to the home screen after logging in correctly. We also test the ability to log out by pretending to log out and making sure that the user is taken back to the login screen.
Conclusion
Mastering the art of writing thorough test cases is essential for making great apps in the fast-paced world of Flutter development. Developers can make sure their Flutter apps don’t have bugs by following the testing guidelines and being good at using widget, unit, and integration tests. Let’s move forward with trust, making testing a natural part of our development process and starting a new era of flawless Flutter apps.
Author Bio:
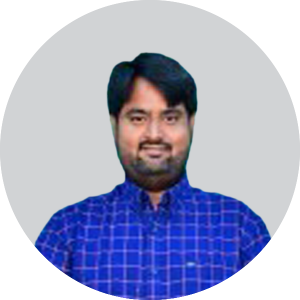
Kesava KANTIPUDI
Mobility - Associate Manager
I am a seasoned software engineer with a passion for mobile app development. With over four years of experience in Flutter, I have honed my skills in creating robust and user-friendly applications. My expertise in Flutter testing ensures that apps not only look great but also perform reliably across different devices and scenarios. I am dedicated to sharing my knowledge and insights to help fellow developers excel in their app development journey.