Purpose of the Article: To explain a few GoLang programs.
Intended Audience: Everyone who is new to Go Programming Language.
Tools and Technology: Visual Studio Code and GOLang.
Keywords: GO, GOLang, .go, Programs, Palindrome, Reverse a given string.
Programming Knowledge on GOLang
The Programs that I am going to discuss are:
- How to check if the given Input is an Alphabet or a Number?
- How to remove spaces form a string?
- How to check if a series is a Palindrome or not?
- Write a Program to check if the series contains a number, special character, and alphabet.
Before writing the code, it is necessary to install Golang on the system or computer, for which the most appropriate source has been the official Golang’s website wherein the latest stable version of Golang is available.
Curious as to why we only refer to the latest and stable-most version if you are a beginner. So, the issue with Programming languages is that they continually get updated with new additional features or functions with improvements in their performance or bug fixes.
These things are usually seen in every update, but are unknown to the future. I guess it would be something different.
What About Stable Then?
Nightly Builds are the most recent with the latest development , but will have many bugs and ongoing testing functions. So, if you are a developer tester or keen to know the features, go ahead. Now, as recommended let’s dive into the topic. Programming is an art! let’s learn together and enjoy the process.
Initially, the file was downloaded and executed (Using the administrator privilege). Installed within a few minutes it already set a path in the environment variable. Unlike other programming languages (C, C++, Java, Python), it had done its job automatically.
After which, a check was conducted using the go version command in the terminal to verify if Golang was installed or not. It showed the version go1.17.6 windows/amd64.
In case of any difficulties in installation process, go to the Golang Official Installation Document Website. Each step has been mentioned clearly, including the installation process for Linux or any other machines.
With this, the installation of Golang in your system is complete.
Moving on to step two: Install visual studio code. In order to write a code, you could use any other IDE (INTEGRATED DEVELOPMENT ENVIRONMENT) or even a Text editor. If an IDE is used, do not forget to download its plugin or extension for Golang from its marketplace. In this case, GO has been downloaded from GO From GOOGLE Extension.
Next, open the Command Palette by right-clicking on the screen, or you can even use the ctrl+shift+p shortcut key to open it. Then after the arrow, type > Go:
- Install / Update Tool.
- Make sure all the checkboxes are ticked.
- Find attached, a snap of what it would look like on the next page.
- After this, click on the Ok tab, which is present on the right.
A terminal will open at the bottom and will do its operation.
With this, the installation of go tools is done.
These tools come in handy when writing the code and provide shortcuts helping us know which function does what in its description.
Now, let’s code !
Before solving the code, I created a folder named Assessment_And_Task/First_Task.
- How to check if the input is an alphabet or a number?
First, create a new file in the IDE and name it check_if_its_alphabet_or_number.go in my case. The “.go” is the extension we use for Golang.
I have, attached codes in the form of a picture for every question as a reference.
Got a question about what a package is, import fmt, func, etc.?
The package is used to create a package that contains many programs with many functions.
Fmt is also a package. That is why we are importing it. In fmt, we have used the Println function, and we have used it in our process. Similarly, we are also creating a package. Let’s talk about func main. We are using the primary function, and the code should start running from here like any other programming language. Now, let’s talk about the program.
Coming to its working and logic, I had created the program so that it would only accept inputs from users in the form of strings. If the user even gives a unique character or number, it would only take it as a string. I stored the input provided by the user in a variable, and then used the concept of maps, checking each character in the string, whether a number or an alphabet or if it’s something other than those.
Firstly, I used the blank identifier “_” as the variable for key-value as I don’t need its value for the current program. A Blank Identifier means ignoring the value. Then I took the value for that key in the variable my_character. This my_character will have the Unicode values for a single character of the string as the for loop runs. This loop runs for the range of my_character. It will run for the string length. So, now using the IsNumber function of the Unicode package, shall check if the Unicode is for a number or not. If it belongs to it, it should convert the Unicode into a string, print it in the console, and state that it is a number.
Similarly, using the IsAlphabet function, I have done it for the alphabet. If both conditions fail, it should print that it is neither an alphabet nor a number.
For initial testing purposes, I gave a default input and took one as the variable for a key-value to test how the code works. You can use them to learn more about the functionalities.
After writing the program, open the terminal and go to the location where this file primary.go was present and write the command go run main.go after hitting this command. Entering the input, and the given information, will show whether the individual character in the string is an alphabet a number or some other character. Yes, we can do it even for a single character, but it gets simple when we know the type of character.
- How to Remove Spaces for a string?
Now, we need to create a new file with the name remove_space.go in my case and write the code. Code is just present after the explanation.
While writing this code, the main issue taking in the input was that the scan function did not accept white spaces. So, to take the information, I needed to learn about this bufio package and NewReader, ReadString role in that package. Here the program will take the input until the user hits enter key; that is why I have used the \n or newline escape sequence. It is like a condition or delimiter. But because of using it, the input considers the condition as the input. So, whatever the information provided at the end contains the delimiter characters.
The program needed to remove the space if the string contained the space character. So, for that condition, I used the if-else statement. It was done, but the main disadvantage of this program was that I needed to create another variable. It would cost memory in the system. We can go with trim or replace functions of the strings package, but one should try to do the program without using the inbuilt package.
This is how I made the program and the problem that I faced. I always debug the code to check cases. Like here, the key value was acting weirdly. So, I needed to initialize the I variable separately.
Here is the code.
How to check if the string is a Palindrome?
Again, create a file with the name palindrome.
Refer to the code below:
Each case worked, but while developing the initial case of storing the value, there was a problem with the fundamental value. For a standard string, the values needed to be from 0, then one, then two, etc. For reverse, it should be the reverse which means it should start with the last value of the regular string and end with the first value. Confused? It is simple if you know what a palindrome is. I made the last value the first value and so on. If the standard and reverse strings are the same, it is a palindrome.
For example, if you reverse the word mom, it will be read as ‘mom’ again. That is what we call a palindrome.
The concept is simple:
- Just reverse the string.
- Store it.
- Check if the input string is equal to the reversed string.
The job is done. But it would help if you were good with maps and reversing things.
- Write a Program to check if the string contains a Number, Special Character, and Alphabet.
Again, create a file with the name.
Check_if_it_contains_alphabet_number_specialCharacter.go in this case and write the code.
Where’s the code?
You will find the code after the explanation.
Finally, for the last program, as I got familiar with Golang by now.
I took the first program and created the same here. Then searched for a unique character and found a simple tip: these special characters were continuous and had some range. So, taking this advantage I created an if-else condition before the else condition saying it was the particular character condition.
Then I created a condition in a way that if for each condition the character can satisfy the specific situation, it should return true for that particular character. So, if the string contains an alphabet, a number, and a series, it should say that it has all the specific conditions, specify what condition it needs.
Finally created a password that contains an alphabet, a number, and a unique character.
Below is the code that I created by me, that can be used as a referred.
Always try to debug and understand the code. It would always lead you to find the root cause of the error. Errors are not always made; at times they occur from the programs themselves.
Thank you for reading my article.
Author Bio:
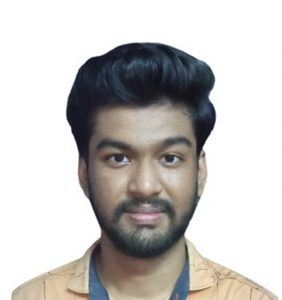
Sudeb Dolui
Cloud Solutions - Digital Transformation
I am an Associate Software Engineer from cloud solutions digital transformation team at Mouri Tech. Currently I am working as A Front-End Developer in a Project. I am a Tech Enthusiast and like to explore and learn new Technologies that are evolving. I have an experience of 1 Year in this field.